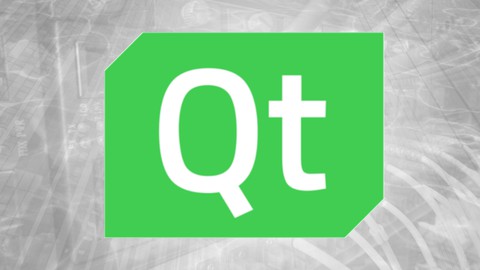
Qt 5 Design Patterns
Qt 5 Design Patterns with C++ For The Advanced Developer
What you’ll learn
-
Design patterns using Qt 5 and C++
-
Creational patterns
-
Structural patterns
-
Behavioral patterns
-
IO Patterns
-
State patterns
-
State machine framework
-
Abstract Factory
-
Builder
-
Factory Method
-
Object Pool
-
Prototype
-
Magic Static
-
Adapter
-
Bridge
-
Composite
-
Decorator
-
Facade
-
Flyweight
-
Chain of responsibility
-
Command
-
Interpreter
-
Iterator
-
Mediator
-
Memento
-
Null Object
-
Strategy
-
Visitor
-
Templates
-
Class construction
-
Memory managment
-
Smart pointers
-
Encapsulation
-
Qt property system
-
SOLID – Single-responsibility Principle
-
SOLID – Open-closed Principle
-
SOLID – Liskov substitution principle
-
SOLID – Interface segregation principle
-
SOLID – Dependency Inversion principle
-
DRY – Don’t repeat yourself
-
KIS – Keep it simple
-
RAII – Resource Acquisition Is Initialization
-
Exception handling
-
Auto connecting signals and slots
-
Threading
-
Thread Pools
-
Qt Concurrent
-
Threading in Widgets
-
Threading in QML
-
Templates
-
Qt Template classes
-
Pointers in templates
-
QMake tricks
-
CMake tricks
-
Creating shared libraries
-
Using shared libraries
-
Loading shared libraries dynamically
-
Creating plugins
-
Loading plugins
-
Text Streams
-
Data Streams
-
Data Classes
-
Storing passwords
-
Controlling processes
-
Desktop services
-
QProcess in Widgets
-
QProcess in QML
-
Signal connection types
-
Disconnecting signals
-
Serializing objects
-
QML state
Requirements
-
Qt Core beginners with Qt 5
-
Qt Core intermediate with Qt 5
-
Qt Core Advanced with Qt 5
-
Qt Widgets beginners with Qt 5
-
QML beginners with Qt 5
-
Advanced level knowledge and experience with Qt
-
Advanced level knowledge and experience with C++
Who this course is for:
- Advanced Qt C++ developers